TypeScript is one of the most popular scripting languages and my favorite web language too, that provides great power (types) to JavaScript and makes it a strictly typed language. Generics is one of my favorite features of TypeScript. Generic types are amazing to increase the flexibility of a function or class or an interface in Typescript, and I never miss a chance to use generic wherever I can. Almost every generic piece of code uses Generic Types, but at the same time, it also restricts the user in some way. Here in this article, I will walk you through how you can make generic types optional in TypeScript so that you can use it without forced to pass a generic type.
Related Article: Why you should learn TypeScript and ditch JavaScript? TypeScript vs JavaScript
Make typescript generic type optional: Quick Solutions
The way we give a default value to a parameter of a function to make it optional, the similar way we do for the generics.
To make a generic type optional, you have to assign the void as the default value.
In the example below, even though the function takes a generic type T, still you can call this function without passing the generic type and it takes void as default.
function fetchData <T = void> ( url: string): T {
const response:T = fetch(url);
return response
}
Now you can pass the fetchData
either passing generic type or without it.
interface User {
id: string;
name: string;
}
// Function call with passing generic type, to fetch the user and return the user, whose type is passed as a generic type.
fetchData<User>('users/1');
// Function call without passing generic type, just to register the device, that doesn't return anything.
fetchData('register-device');
Detailed Example of optional generic type
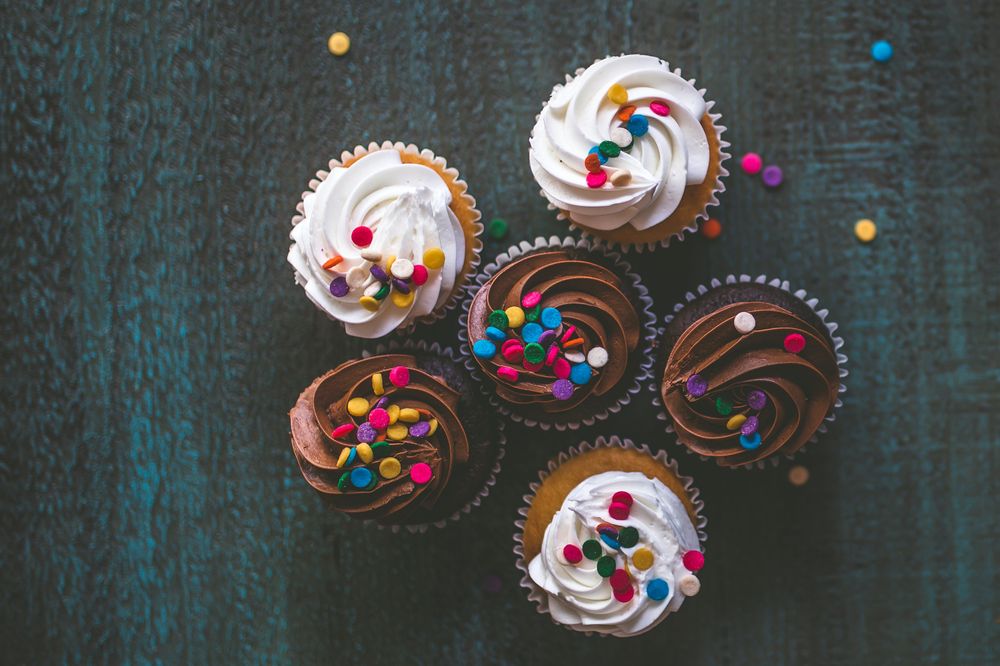
Generic types can be more complicated when passing from one type to another and making optional into one and required in another. In the example below, I've created a type for a function BaseFunctionType
and another type FunctionType
that uses BaseFunctionType
. In the BaseFunctionType
are the two parameters where both are required, now we want to use that type in a function without passing the second parameter, so FunctionType
is created to pass the second generic type as void and make it optional for the function. Now we assign the FunctionType
to our someFunction
and pass a single parameter.
type BaseFunctionType<T1, T2> = (a:T1, b:T2) => void;
type FunctionType<T = void> = BaseFunctionType<{name: string}, T>
const someFunction:FunctionType = (a) => {
}
someFunction({ name: "Siraj" })
Method 2: With function overloading
You can also make a generic optional using two function declarations, one with a single generic and two generics. So when calling with a single generic, it will automatically pick the first declaration and not expect the second generic type. Similarly, if you pass the second generic parameter, it will pick the second function declaration. This method is not much of use, as it only works on functions mainly. See the codes below.
class Logger {
// Declarations
private logData<T>(operation: string, responseData: T):T
private logData<T, S>(operation: string, responseData: T, requestData?: S):T
// Definition
private logData<T, S>(operation: string, responseData: T, requestData?: S) {
// ...body
}
}
If that doesn't fit your case, don't worry, the quick solution is universal and works in any case.
Frequently asked questions.
What is TypeScript?
TypeScript is an open-source programming language developed by Microsoft. It is the superset of JavaScript that provides types and some additional features to JavaScript. You can also call it strictly typed JavaScript.
Should I use TypeScript?
If you use JavaScript and still wondering if you should TypeScript, then YES!!, you must give it a try. Here you can read why you should learn TypeScript over JavaScript.
What are generics in TypeScript?
Generic is a feature in the language TypeScript to make the module (interface, function, etc.) more generic. But does it do it? Suppose you call a function that returns something, but the type of the return changes based on the type in the parameter, so it changes on every call to call. So it is not possible to hardcode a type in the function return type, so what would we do in that case? Use `any`? No! Giving any to the return type doesn't contain any info about the type. So the returned value from the function is always unknown, so it is not actually dependent on the type of function parameter.
Does ReactJS use TypeScript?
ReactJS is written in JavaScript, but at the same time, it provides support for TypeScript. You can use create-react-app for typescript. Even this site is developed in TypeScript.